Item Appraisal
Version: 1.1
Author: SeMcDun
Date: 2015.01.02
Version History
- <Version 1.1> 2015.01.04 - Bug Fix: Drawing a skill's name would break the game.
- <Version 1.0> 2015.01.02 - Original Release
Description
The general idea is; Items must be appraised before their details can be visible.
Items that have not been appraised cannot be equipped and will appear as "? ? ? ? ?" in the inventory.
Features
- Choose how much or how little is hidden from the player. (Item name, description, icon)
- Choose the substitute text for the item name and description.
- Items can be appraised from a shop styled scene or by using items.
- Purchasing unappraised items from the shop can appraise the item.
- Use the script call appraise to load the shop type scene.
- At the moment, the cost to appraise an item from the shop is just the cost of the item itself.
ScreenshotsThe Inventory
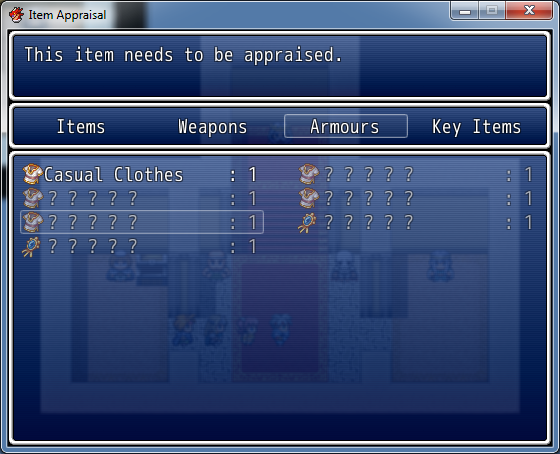
When an item is appraised...

Using an appraising item from the menu...

Displays the number of appraisal item uses left

Instructions
Add the notetag <appraise> to weapons and armour. This makes them need to be appraised.
Add the notetag <appraise> to items to make them appraising items.
Script
#==============================================================================
# * Item Appraisal VXA v1.1
# * -- By: SeMcDun
# * -- Last Updated: 2015.01.04
#------------------------------------------------------------------------------
# Description:
# Certain equipment can only be equipped once they have been appraised.
# Items that are not appraised can have their name, icon and/or description
# hidden.
#------------------------------------------------------------------------------
# Installation:
#
# Weapon/Armor Notetags;
#-----------------------
# <appraise>
# This means the weapon/armor must be appraised before its details are shown.
#
# Script Calls;
#--------------
# appraise
# This calls the appraise shop scene.
#
# appraise_from_item
# This calls the appraise from the inventory scene.
#==============================================================================
# * Import
#------------------------------------------------------------------------------
$imported = {} if $imported.nil?
$imported["SEM-item_appraisal"] = true
#==============================================================================
# * module SEM
#------------------------------------------------------------------------------
module SEM
module REGEXP
ITEM_APPRAISAL = /<appraise>/i
end
module ITEM_APPRAISAL
# The item name displayed when hidden
UNAPPRAISED_TEXT = "? ? ? ? ?"
# The description displayed when hidden
UNAPPRAISED_DESCRIPTION = "This item needs to be appraised."
# When an item is displayed, this text is shown after the item name
APPRAISED_RESULT = " was appraised!"
# When using an item to appraise, this is displayed after the number of
# items left.
ITEMS_LEFT_TEXT = "Uses"
# Turn on/off what is hidden/shown before appraisal.
SHOW_DESCRIPTION = false
SHOW_NAME = false
SHOW_ICON = true
# This is for compatibility reasons
# If you use a script which edits the BattleManager gain_drop_items function
# then this needs to be false. And you'll need a work around if you want
# battle drops to appear unappraised.
OVERWRITE_DROPS = true
# Set whether or not items purchased at a store are auto appraised
AUTO_APPRAISE_PURCHASED = true
# Whether or not to overwrite shop methods
OVERWRITE_SHOPS = true
# Hide the stat bonus in shops?
HIDE_SHOP_STATS = true
# Hide the number of items possessed in shops when not appraised?
# This means the player won't know if they have the item in their inventory
# or not when purchasing unappraised items from the shop.
HIDE_NUMBER_POSSESSED = true
# The text to be displayed in a shop when stats are hidden.
STORE_STAT = "???"
end
end
#==============================================================================
# ■ DataManager
#==============================================================================
module DataManager
#--------------------------------------------------------------------------
# alias method: load_database
#--------------------------------------------------------------------------
class <<self; alias load_database_itemappraisal load_database; end
def self.load_database
load_database_itemappraisal
notetags_itemappraisal
end
#--------------------------------------------------------------------------
# new method: notetags_itemappraisal
#--------------------------------------------------------------------------
def self.notetags_itemappraisal
for weapon in $data_weapons
next if weapon.nil?
weapon.load_notetags_itemappraisal
end
for armor in $data_armors
next if armor.nil?
armor.load_notetags_itemappraisal
end
for item in $data_items
next if item.nil?
item.load_notetags_itemappraisal
end
end
end
#==============================================================================
# * RPG::EquipItem
#==============================================================================
class RPG::EquipItem < RPG::BaseItem
def load_notetags_itemappraisal
# @appraised begins as true so only items that are TAGGED become hidden
@appraised = true
self.note.split(/[\r\n]+/).each { |line|
case line
#---
when SEM::REGEXP::ITEM_APPRAISAL
@appraised = false
#---
end
} # self.note.split
#---
end
def appraised?
return @appraised
end
def appraise_item
@appraised = true
end
def appraisal_item?
# Weapons/Armour can't be an appraisal item
return false
end
end
#==============================================================================
# * RPG::Item
#==============================================================================
class RPG::Item < RPG::UsableItem
def load_notetags_itemappraisal
# @appraised begins as true so only items that are TAGGED become hidden
@appraisal_item = false
self.note.split(/[\r\n]+/).each { |line|
case line
#---
when SEM::REGEXP::ITEM_APPRAISAL
@appraisal_item = true
#---
end
} # self.note.split
#---
end
def appraisal_item?
return @appraisal_item
end
def appraised?
# Items aren't able to be appraised, so they are automatically visible
return true
end
end
#==============================================================================
# ** Window_EquipItem
#------------------------------------------------------------------------------
# This window displays choices when opting to change equipment on the
# equipment screen.
#==============================================================================
class Window_EquipItem < Window_ItemList
#--------------------------------------------------------------------------
# * Include in Item List?
#--------------------------------------------------------------------------
alias window_equipitem_include_sem_ia include?
def include?(item)
if item != nil
return false if item.appraised? == false
end
window_equipitem_include_sem_ia(item)
end
end
#==============================================================================
# * RPG::UsableItem
#==============================================================================
class RPG::UsableItem
def appraised?
# UsableItems aren't able to be appraised, so they are automatically visible
return true
end
end
#==============================================================================
# ** Window_Base
#------------------------------------------------------------------------------
# This is a super class of all windows within the game.
#==============================================================================
class Window_Base < Window
#--------------------------------------------------------------------------
# * Draw Item Name
# enabled : Enabled flag. When false, draw semi-transparently.
#--------------------------------------------------------------------------
alias window_base_draw_item_name_sem_ia draw_item_name
def draw_item_name(item, x, y, enabled = true, width = 172)
# Do the appraisal check
return unless item
if item.appraised? == false
# If the item is not appraised
if SEM::ITEM_APPRAISAL::SHOW_ICON
draw_icon(item.icon_index, x, y, enabled)
end
change_color(normal_color, enabled)
if SEM::ITEM_APPRAISAL::SHOW_NAME
draw_text(x + 28, y, width, line_height, item.name, 0)
else
draw_text(x + 28, y, width, line_height, SEM::ITEM_APPRAISAL::UNAPPRAISED_TEXT, 0)
end
else
# Item is appraised - or doesn't need to be
window_base_draw_item_name_sem_ia(item, x, y, enabled = true, width = 172)
end
end
end #
#==============================================================================
# ** Window_Help
#------------------------------------------------------------------------------
# This window shows skill and item explanations along with actor status.
#==============================================================================
class Window_Help < Window_Base
#--------------------------------------------------------------------------
# * Set Item
# item : Skills and items etc.
#--------------------------------------------------------------------------
def set_item(item)
if item.is_a?(RPG::Weapon) || item.is_a?(RPG::Armor)
# Weapon or Armour - Check whether to show a description or not
if item.appraised? == true
# Appraised - Show description
set_text(item ? item.description : "")
else
# Not appraised, check whether to show the description or not
if SEM::ITEM_APPRAISAL::SHOW_DESCRIPTION
set_text(item ? item.description : "")
else
set_text(item ? SEM::ITEM_APPRAISAL::UNAPPRAISED_DESCRIPTION : "")
end
end
else
# Is not a weapon or armour
set_text(item ? item.description : "")
end
end
end
#==============================================================================
# ** Scene_AppraiseItem
#==============================================================================
class Scene_AppraiseItem < Scene_Item
#--------------------------------------------------------------------------
# * Start Processing
#--------------------------------------------------------------------------
def start
super
create_help_window
create_category_window
create_item_window
create_result_window
create_gold_window
end
#--------------------------------------------------------------------------
# * Create Gold Window
#--------------------------------------------------------------------------
def create_gold_window
@gold_window = Window_Gold.new
@gold_window.x = (Graphics.width - 160)
@gold_window.y = @help_window.height
end
#--------------------------------------------------------------------------
# * Create Item Window
#--------------------------------------------------------------------------
def create_item_window
wy = @category_window.y + @category_window.height
wh = Graphics.height - wy
@item_window = Window_AppraiseItemList.new(0, wy, Graphics.width, wh)
@item_window.viewport = @viewport
@item_window.help_window = @help_window
@item_window.set_handler(:ok, method(:on_item_ok))
@item_window.set_handler(:cancel, method(:on_item_cancel))
@category_window.item_window = @item_window
end
#--------------------------------------------------------------------------
# * Create Category Window
#--------------------------------------------------------------------------
def create_category_window
@category_window = Window_AppraiseItemCategory.new
@category_window.viewport = @viewport
@category_window.help_window = @help_window
@category_window.y = @help_window.height
@category_window.set_handler(:ok, method(:on_category_ok))
@category_window.set_handler(:cancel, method(:return_scene))
end
def create_result_window
w = (Graphics.width / 3) * 2
h = 48
x = (Graphics.width / 2) - (w / 2)
y = (Graphics.height / 2) - (h / 2)
@result_window = Window_AppraisalResult.new(x,y,w,h)
@result_window.set_handler(:ok, method(:on_result_ok))
@result_window.set_handler(:cancel, method(:on_result_cancel))
end
def on_result_ok
hide_result
activate_item
end
def on_result_cancel
hide_result
activate_item
end
def hide_result
@result_window.deactivate
@result_window.unselect
@result_window.hide
end
def activate_item
@item_window.activate
@item_window.select(0)
end
#--------------------------------------------------------------------------
# * Item [OK]
#--------------------------------------------------------------------------
def on_item_ok
item.appraise_item
$game_party.lose_gold(item_appraise_cost(item))
@result_window.show
@result_window.activate
@result_window.select(0)
@result_window.set_text(item)
refresh_windows
end
def item_appraise_cost(item)
return item.price
end
def refresh_windows
@item_window.refresh
@gold_window.refresh
end
end
#==============================================================================
# ** Window_AppraiseItemCategory
#==============================================================================
class Window_AppraiseItemCategory < Window_ItemCategory
#--------------------------------------------------------------------------
# * Get Digit Count
#--------------------------------------------------------------------------
def col_max
return 2
end
#--------------------------------------------------------------------------
# * Create Command List
#--------------------------------------------------------------------------
def make_command_list
add_command(Vocab::weapon, :weapon)
add_command(Vocab::armor, :armor)
end
#--------------------------------------------------------------------------
# * Get Window Width
#--------------------------------------------------------------------------
def window_width
return (Graphics.width - 160)
end
end
#==============================================================================
# ** Window_ItemList
#------------------------------------------------------------------------------
# This window displays a list of party items on the item screen.
#==============================================================================
class Window_ItemList < Window_Selectable
#--------------------------------------------------------------------------
# * Create Item List
#--------------------------------------------------------------------------
def make_item_list
@data = $game_party.all_items.select {|item| include?(item) }
@data += $game_party.all_items.select {|item| include_appraised?(item) }
@data.push(nil) if include?(nil)
end
#--------------------------------------------------------------------------
# * Include in Item List?
#--------------------------------------------------------------------------
def include?(item)
case @category
when :item
item.is_a?(RPG::Item) && !item.key_item?
when :weapon
item.is_a?(RPG::Weapon) && item.appraised?
when :armor
item.is_a?(RPG::Armor) && item.appraised?
when :key_item
item.is_a?(RPG::Item) && item.key_item?
else
false
end
end
#--------------------------------------------------------------------------
# * Include in Item List?
#--------------------------------------------------------------------------
def include_appraised?(item)
case @category
when :item
item.is_a?(RPG::Item) && !item.key_item? && !@data.include?(item)
when :weapon
item.is_a?(RPG::Weapon) && !item.appraised? && !@data.include?(item)
when :armor
item.is_a?(RPG::Armor) && !item.appraised? && !@data.include?(item)
when :key_item
item.is_a?(RPG::Item) && item.key_item? && !@data.include?(item)
else
false
end
end
end
#==============================================================================
# ** Window_AppraiseItemList
#------------------------------------------------------------------------------
# This window displays a list of party items on the item screen.
#==============================================================================
class Window_AppraiseItemList < Window_ItemList
#--------------------------------------------------------------------------
# * Include in Item List?
#--------------------------------------------------------------------------
def include?(item)
return false
end
#--------------------------------------------------------------------------
# * Display in Enabled State?
#--------------------------------------------------------------------------
def enable?(item)
return false if item == nil
if $game_party.gold >= item_appraise_cost(item)
return true
else
return false
end
end
#--------------------------------------------------------------------------
# * Draw Number of Items
#--------------------------------------------------------------------------
def draw_item_number(rect, item)
# Instead of drawing the item number - draw the appraisal cost
draw_text(rect, sprintf("%2d" + Vocab::currency_unit, item_appraise_cost(item)), 2)
end
def item_appraise_cost(item)
return item.price
end
end
#==============================================================================
# ** Window_Base
#------------------------------------------------------------------------------
# This is a super class of all windows within the game.
#==============================================================================
class Window_AppraisalResult < Window_Selectable
#--------------------------------------------------------------------------
# * Object Initialization
#-------------------------------------------------------------------------
def initialize(x, y, width, height)
super
@index = -1
self.z += 100
@handler = {}
@cursor_fix = false
@cursor_all = false
update_padding
deactivate
hide
end
def set_text(item)
contents.clear
draw_icon(item.icon_index, 4, 0, true)
draw_text(32, 0, width - 24 - 32, 24, item.name + SEM::ITEM_APPRAISAL::APPRAISED_RESULT, 1)
end
end
#==============================================================================
# ** Game_Interpreter
#------------------------------------------------------------------------------
# An interpreter for executing event commands. This class is used within the
# Game_Map, Game_Troop, and Game_Event classes.
#==============================================================================
class Game_Interpreter
def appraise
SceneManager.call(Scene_AppraiseItem)
end
def appraise_from_item
SceneManager.call(Scene_ItemMenuAppraise)
end
end
#==============================================================================
# ** Scene_Item
#------------------------------------------------------------------------------
# This class performs the item screen processing.
#==============================================================================
class Scene_Item < Scene_ItemBase
#--------------------------------------------------------------------------
# * Item [OK]
#--------------------------------------------------------------------------
alias scene_item_on_item_ok_sem_ia on_item_ok
def on_item_ok
$game_temp.item_menu_appraise_item = nil
if item.appraisal_item?
$game_temp.item_menu_appraise_item = item
SceneManager.call(Scene_ItemMenuAppraise)
else
scene_item_on_item_ok_sem_ia
end
end
end
#==============================================================================
# ** Scene_ItemMenuAppraise
#==============================================================================
class Scene_ItemMenuAppraise < Scene_Item
#--------------------------------------------------------------------------
# * Start Processing
#--------------------------------------------------------------------------
def start
super
create_help_window
create_category_window
create_item_window
create_result_window
create_qty_window
end
def create_qty_window
w = 160
h = 48
x = Graphics.width - 160
y = 48 + 24
@qty_window = Window_AppraiseQty.new(x,y,w,h)
@qty_window.refresh
end
#--------------------------------------------------------------------------
# * Create Item Window
#--------------------------------------------------------------------------
def create_item_window
wy = @category_window.y + @category_window.height
wh = Graphics.height - wy
@item_window = Window_ItemMenuAppraiseItemList.new(0, wy, Graphics.width, wh)
@item_window.viewport = Viewport.new
@item_window.help_window = @help_window
@item_window.set_handler(:ok, method(:on_item_ok))
@item_window.set_handler(:cancel, method(:on_item_cancel))
@category_window.item_window = @item_window
end
#--------------------------------------------------------------------------
# * Create Category Window
#--------------------------------------------------------------------------
def create_category_window
@category_window = Window_AppraiseItemCategory.new
@category_window.viewport = Viewport.new
@category_window.help_window = @help_window
@category_window.y = @help_window.height
@category_window.set_handler(:ok, method(:on_category_ok))
@category_window.set_handler(:cancel, method(:return_scene))
end
def create_result_window
w = (Graphics.width / 3) * 2
h = 48
x = (Graphics.width / 2) - (w / 2)
y = (Graphics.height / 2) - (h / 2)
@result_window = Window_AppraisalResult.new(x,y,w,h)
@result_window.set_handler(:ok, method(:on_result_ok))
@result_window.set_handler(:cancel, method(:on_result_cancel))
end
def on_result_ok
hide_result
# If the number of appraising items reaches 0, return scene
if $game_party.item_number($game_temp.item_menu_appraise_item) <= 0
return_scene
end
activate_item
end
def on_result_cancel
hide_result
activate_item
end
def hide_result
@result_window.deactivate
@result_window.unselect
@result_window.hide
end
def activate_item
@item_window.activate
@item_window.select(0)
end
#--------------------------------------------------------------------------
# * Item [OK]
#--------------------------------------------------------------------------
def on_item_ok
item.appraise_item
$game_party.lose_item($game_temp.item_menu_appraise_item, 1)
@result_window.show
@result_window.activate
@result_window.select(0)
@result_window.set_text(item)
refresh_windows
end
def item_appraise_cost(item)
return 1
end
def refresh_windows
@item_window.refresh
@qty_window.refresh
end
end
#==============================================================================
# ** Window_AppraiseQty
#------------------------------------------------------------------------------
# This is a super class of all windows within the game.
#==============================================================================
class Window_AppraiseQty < Window_Base
def refresh
contents.clear
if $game_temp.item_menu_appraise_item
draw_text(0, 0, width - 24, 24, $game_party.item_number($game_temp.item_menu_appraise_item).to_s + " " + SEM::ITEM_APPRAISAL::ITEMS_LEFT_TEXT, 2)
else
draw_text(0, 0, width - 24, 24, "Error: Please Report", 2)
end
end
end
#==============================================================================
# ** Game_Temp
#------------------------------------------------------------------------------
# This class handles temporary data that is not included with save data.
# The instance of this class is referenced by $game_temp.
#==============================================================================
class Game_Temp
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_accessor :item_menu_appraise_item
#--------------------------------------------------------------------------
# * Alias: Object Initialization
#--------------------------------------------------------------------------
alias gt_init_sem_ia initialize
def initialize
gt_init_sem_ia
@item_menu_appraise_item = nil
end
end
#==============================================================================
# ** Window_ItemMenuAppraiseItemList
#------------------------------------------------------------------------------
# This window displays a list of party items on the item screen.
#==============================================================================
class Window_ItemMenuAppraiseItemList < Window_ItemList
#--------------------------------------------------------------------------
# * Include in Item List?
#--------------------------------------------------------------------------
def include?(item)
return false
end
#--------------------------------------------------------------------------
# * Display in Enabled State?
#--------------------------------------------------------------------------
def enable?(item)
return false if item == nil
return true
end
#--------------------------------------------------------------------------
# * Draw Number of Items
#--------------------------------------------------------------------------
def draw_item_number(rect, item)
# Instead of drawing the item number - draw the appraisal cost
end
def item_appraise_cost(item)
return item.price
end
end
#==============================================================================
# ** BattleManager
#------------------------------------------------------------------------------
# This module manages battle progress.
#==============================================================================
module BattleManager
if SEM::ITEM_APPRAISAL::OVERWRITE_DROPS
#--------------------------------------------------------------------------
# * overwrite: Dropped Item Acquisition and Display
#--------------------------------------------------------------------------
def self.gain_drop_items
$game_troop.make_drop_items.each do |item|
$game_party.gain_item(item, 1)
if item.appraised?
item_name = item.name
else
item_name = SEM::ITEM_APPRAISAL::UNAPPRAISED_TEXT
end
$game_message.add(sprintf(Vocab::ObtainItem, item_name))
end
wait_for_message
end
end
end
#==============================================================================
# ** Scene_Shop
#------------------------------------------------------------------------------
# This class performs shop screen processing.
#==============================================================================
class Scene_Shop < Scene_MenuBase
#--------------------------------------------------------------------------
# * Execute Purchase
#--------------------------------------------------------------------------
alias scene_shop_do_buy_sem_ia do_buy
def do_buy(number)
scene_shop_do_buy_sem_ia(number)
if SEM::ITEM_APPRAISAL::AUTO_APPRAISE_PURCHASED
@item.appraise_item
end
end
end
#==============================================================================
# ** Window_ShopStatus
#------------------------------------------------------------------------------
# This window displays number of items in possession and the actor's
# equipment on the shop screen.
#==============================================================================
class Window_ShopStatus < Window_Base
if SEM::ITEM_APPRAISAL::OVERWRITE_SHOPS
#--------------------------------------------------------------------------
# * overwrite: Draw Actor Equipment Information
#--------------------------------------------------------------------------
alias window_shopstatus_dae_sem_ia draw_actor_equip_info
def draw_actor_equip_info(x, y, actor)
if SEM::ITEM_APPRAISAL::HIDE_SHOP_STATS && !@item.appraised?
enabled = actor.equippable?(@item)
change_color(normal_color, enabled)
draw_text(x, y, 112, line_height, actor.name)
item1 = current_equipped_item(actor, @item.etype_id)
draw_appraisal_stat if enabled
draw_item_name(item1, x, y + line_height, enabled)
else
window_shopstatus_dae_sem_ia(x, y, actor)
end
end
def draw_appraisal_stat
end
#--------------------------------------------------------------------------
# * overwrite: Draw Quantity Possessed
#--------------------------------------------------------------------------
def draw_possession(x, y)
rect = Rect.new(x, y, contents.width - 4 - x, line_height)
change_color(system_color)
draw_text(rect, Vocab::Possession)
change_color(normal_color)
if SEM::ITEM_APPRAISAL::HIDE_NUMBER_POSSESSED
if @item
if @item.appraised?
draw_text(rect, $game_party.item_number(@item), 2)
end
else
draw_text(rect, $game_party.item_number(@item), 2)
end
else
draw_text(rect, $game_party.item_number(@item), 2)
end
end
end
end
Credit
Support
Post any problems here and I'll try to fix them as soon as possible.
Known Compatibility Issues
This script overwrites parts of the shop and battlemanager (drops method) but this can be turned off in the script.
Demo
See Attached.
Author's Notes
First script of the new year. I wish everyone a productive year!
Terms of Use
Free for non-commercial projects.