Simplistic Single-Player CMS (VXA)
Version: 1.0
Author: Zylos
Date: May 11, 2012
Description
This script replaces the default menu system with a much simpler menu designed for single player games (i.e. games with only one playable character rather than a full party), although it will function fully with multiple character parties as well if you use the page buttons to tab between characters. The menu simply tracks the player and places itself next to him on the map, removing the status window taking up the whole of the screen. Very simple, decent for non-traditional RPG's.
Features
- Simplifies and removes the clutter of the default menu.
- Optimizes the menu on the map for single player parties.
- Displays the total playtime like it used to in previous RPG Makers.
Screenshots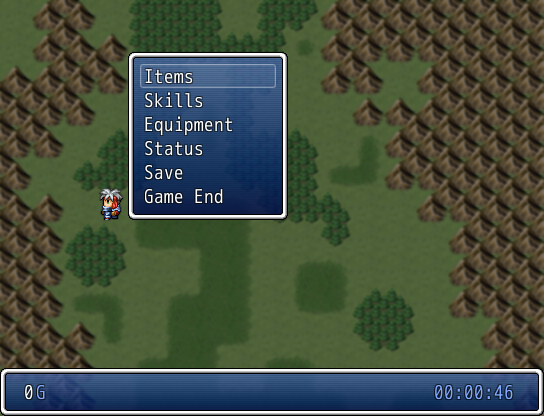
Instructions
- Place this script in the materials section, above Main.
- ?
- ?
- Profit.
Script
#==============================================================================
# Simplistic Single-Player CMS (VXA)
# Version: 1.0
# Author: Zylos (rmrk.net)
# Date: May 11th, 2012
#------------------------------------------------------------------------------
# Description:
#
# This script replaces the default menu system with a much simpler menu
# designed for single player games (i.e. games with only one playable
# character rather than a full party). The menu simply tracks the player
# and places itself next to him, removing the fullscreen status window.
#
#------------------------------------------------------------------------------
# Instructions:
#
# - Place this script in the materials section, above Main.
# - ?
# - ?
# - Profit.
#
#==============================================================================
#==============================================================================
# ** Window_Gold_Time
#------------------------------------------------------------------------------
# This window displays the party's gold and total play time.
#==============================================================================
class Window_GoldTime < Window_Base
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
super(0, 0, window_width, fitting_height(1))
refresh
end
#--------------------------------------------------------------------------
# * Get Window Width
#--------------------------------------------------------------------------
def window_width
return 544
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
contents.clear
draw_currency_value(value, currency_unit, 4, 0, 32)
draw_text(4, 0, 500, line_height, $game_system.playtime_s, 2)
end
#--------------------------------------------------------------------------
# * Get Party Gold
#--------------------------------------------------------------------------
def value
$game_party.gold
end
#--------------------------------------------------------------------------
# Get Currency Unit
#--------------------------------------------------------------------------
def currency_unit
Vocab::currency_unit
end
#--------------------------------------------------------------------------
# * Open Window
#--------------------------------------------------------------------------
def open
refresh
super
end
end
#==============================================================================
# ** Window_MenuCommand
#------------------------------------------------------------------------------
# This command window appears on the menu screen.
#==============================================================================
class Window_MenuCommand < Window_Command
#--------------------------------------------------------------------------
# * Initialize Command Selection Position (Class Method)
#--------------------------------------------------------------------------
def self.init_command_position
@@last_command_symbol = nil
end
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
super(0, 0)
select_last
end
#--------------------------------------------------------------------------
# * Get Window Width
#--------------------------------------------------------------------------
def window_width
return 160
end
#--------------------------------------------------------------------------
# * Get Number of Lines to Show
#--------------------------------------------------------------------------
def visible_line_number
item_max
end
#--------------------------------------------------------------------------
# * Create Command List
#--------------------------------------------------------------------------
def make_command_list
add_main_commands
add_original_commands
add_save_command
add_game_end_command
end
#--------------------------------------------------------------------------
# * Add Main Commands to List
#--------------------------------------------------------------------------
def add_main_commands
add_command(Vocab::item, :item, main_commands_enabled)
add_command(Vocab::skill, :skill, main_commands_enabled)
add_command(Vocab::equip, :equip, main_commands_enabled)
add_command(Vocab::status, :status, main_commands_enabled)
end
#--------------------------------------------------------------------------
# * For Adding Original Commands
#--------------------------------------------------------------------------
def add_original_commands
end
#--------------------------------------------------------------------------
# * Add Save to Command List
#--------------------------------------------------------------------------
def add_save_command
add_command(Vocab::save, :save, save_enabled)
end
#--------------------------------------------------------------------------
# * Add Exit Game to Command List
#--------------------------------------------------------------------------
def add_game_end_command
add_command(Vocab::game_end, :game_end)
end
#--------------------------------------------------------------------------
# * Get Activation State of Main Commands
#--------------------------------------------------------------------------
def main_commands_enabled
$game_party.exists
end
#--------------------------------------------------------------------------
# * Get Activation State of Formation
#--------------------------------------------------------------------------
def formation_enabled
$game_party.members.size >= 2 && !$game_system.formation_disabled
end
#--------------------------------------------------------------------------
# * Get Activation State of Save
#--------------------------------------------------------------------------
def save_enabled
!$game_system.save_disabled
end
#--------------------------------------------------------------------------
# * Processing When OK Button Is Pressed
#--------------------------------------------------------------------------
def process_ok
@@last_command_symbol = current_symbol
super
end
#--------------------------------------------------------------------------
# * Restore Previous Selection Position
#--------------------------------------------------------------------------
def select_last
select_symbol(@@last_command_symbol)
end
end
#==============================================================================
# ** Scene_Menu
#------------------------------------------------------------------------------
# This class performs the menu screen processing.
#==============================================================================
class Scene_Menu < Scene_MenuBase
#--------------------------------------------------------------------------
# * Start Processing
#--------------------------------------------------------------------------
def start
super
create_player_sprite
create_command_window
create_goldtime_window
end
#--------------------------------------------------------------------------
# * Termination Processing
#--------------------------------------------------------------------------
def terminate
super
dispose_background
@player_sprite.dispose
end
#--------------------------------------------------------------------------
# * Frame Update
#--------------------------------------------------------------------------
def update
update_basic
@gold_window.refresh
end
#--------------------------------------------------------------------------
# * Create Player Sprite
#--------------------------------------------------------------------------
def create_player_sprite
@viewport_sprite = Viewport.new
@player_sprite = Sprite_Character.new(@viewport_sprite, $game_player)
@player_sprite.x = $game_player.screen_x
@player_sprite.y = $game_player.screen_y
@player_sprite.z = 1000
end
#--------------------------------------------------------------------------
# * Create Command Window
#--------------------------------------------------------------------------
def create_command_window
@command_window = Window_MenuCommand.new
if $game_player.screen_x < @command_window.width
@command_window.x = $game_player.screen_x + (@player_sprite.width/2)
else
@command_window.x = $game_player.screen_x - (@player_sprite.width/2) - @command_window.width
end
if $game_player.screen_y < @command_window.height
@command_window.y = $game_player.screen_y - @player_sprite.height
else
@command_window.y = $game_player.screen_y-@command_window.height
end
@command_window.set_handler(:item, method(:command_item))
@command_window.set_handler(:skill, method(:command_skill))
@command_window.set_handler(:equip, method(:command_equip))
@command_window.set_handler(:status, method(:command_status))
@command_window.set_handler(:save, method(:command_save))
@command_window.set_handler(:game_end, method(:command_game_end))
@command_window.set_handler(:cancel, method(:return_scene))
end
#--------------------------------------------------------------------------
# * Create Gold_Time Window
#--------------------------------------------------------------------------
def create_goldtime_window
@gold_window = Window_GoldTime.new
@gold_window.x = 0
if $game_player.screen_y < 336
@gold_window.y = Graphics.height - @gold_window.height
else
@gold_window.y = 0
end
end
#--------------------------------------------------------------------------
# * [Item] Command
#--------------------------------------------------------------------------
def command_item
SceneManager.call(Scene_Item)
end
#--------------------------------------------------------------------------
# * [Skill] Command
#--------------------------------------------------------------------------
def command_skill
SceneManager.call(Scene_Skill)
end
#--------------------------------------------------------------------------
# * [Equipment] Command
#--------------------------------------------------------------------------
def command_equip
SceneManager.call(Scene_Equip)
end
#--------------------------------------------------------------------------
# * [Status] Command
#--------------------------------------------------------------------------
def command_status
SceneManager.call(Scene_Status)
end
#--------------------------------------------------------------------------
# * [Save] Command
#--------------------------------------------------------------------------
def command_save
SceneManager.call(Scene_Save)
end
#--------------------------------------------------------------------------
# * [Exit Game] Command
#--------------------------------------------------------------------------
def command_game_end
SceneManager.call(Scene_End)
end
end
Credit