Item Price Adjust
Version: 1.0
Author: JFace
Date: June 19, 2010
Description
This script allows the user to adjust the cost and sale price of items during gameplay. This is particularly useful if using a Haggle type of event wherein the hero can pay less than asking price, and receive more money from sales. The cost and sale adjustments are customizable.
Screenshots
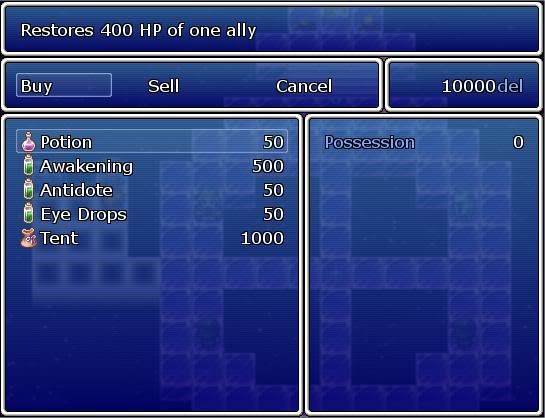
Items at full cost

The same items at half cost

The sale price set at 80%, instead of the default 50%
Instructions
Follow the instructions at the top of the script.
Script
#===============================================================================
#
# Item Price Adjust - By JFace
# July 19, 2010
#
# This script adjusts the cost and sale price of items in game. This is
# particularly useful if using a Haggle type of event wherein the hero can
# pay less than asking price, and receive more money from sales. The cost
# and sale adjustments are customizable.
#
#
#
# Instructions
# -----------------------------------------------------------------------------
# To use this script, place this script anywhere below ? Materials
# but above ? Main. Save your game.
#
# There are four customizable features below. Read the comments.
# ==============================================================================
module JFace
module Discount
#===============================================================================
# Switch Initialization - Set up which switch ID you wish to use. This switch
# controls whether to use the standard item cost (when the switch is [OFF],
# or to use the adjusted item cost (when the switch is [ON].
#
CostSwitch = 3 # <-- This is a switch ID number
#===============================================================================
#===============================================================================
# Cost Adjust Variable - Set up which variable ID you wish to use. This variable
# controls the percent of adjustment to the standard item cost. For instance,
# when the variable value is set to 80, items will cost 80% of standard value.
# The switch associated to CostSwitch must be on for this value to take effect.
#
CostAdjust = 21 # <-- This is a variable ID number
#===============================================================================
#===============================================================================
# Sale Adjust Variable - Set up which variable ID you wish to use. This variable
# controls the percent of adjustment to the standard item sale. For instance,
# when the variable value is set to 50, items will sell for 50% of standard
# value. This value will always be in effect, regardless of CostSwitch.
#
SaleAdjust = 22 # <-- This is a variable ID number
#===============================================================================
#===============================================================================
# Local Switch Initialization - This should generally be kept false.
# false => the CostAdjust variable will not affect the sale price
# true => the CostAdjust variable will affect the sale price
#
DiscountSale = false # <== Must be true or false
#===============================================================================
#===============================================================================
# This concludes the customizable section of this script
#===============================================================================
end
end
#--------------------------------------------------------------------------
# * Draw Item
# index : item number
#--------------------------------------------------------------------------
def draw_item(index)
item = @data[index]
number = $game_party.item_number(item)
if $game_switches[JFace::Discount::CostSwitch] == true
price = item.price*$game_variables[JFace::Discount::CostAdjust]
price = price/100
enabled = (price <= $game_party.gold and number < 99)
rect = item_rect(index)
self.contents.clear_rect(rect)
draw_item_name(item, rect.x, rect.y, enabled)
rect.width -= 4
self.contents.draw_text(rect, price, 2)
else
enabled = (item.price <= $game_party.gold and number < 99)
rect = item_rect(index)
self.contents.clear_rect(rect)
draw_item_name(item, rect.x, rect.y, enabled)
rect.width -= 4
self.contents.draw_text(rect, item.price, 2)
end
end
#==============================================================================
# ** Scene_Shop
#------------------------------------------------------------------------------
# This class performs shop screen processing.
#==============================================================================
class Scene_Shop < Scene_Base
#--------------------------------------------------------------------------
# * Update Buy Item Selection
#--------------------------------------------------------------------------
def update_buy_selection
@status_window.item = @buy_window.item
if Input.trigger?(Input::B)
Sound.play_cancel
@command_window.active = true
@dummy_window.visible = true
@buy_window.active = false
@buy_window.visible = false
@status_window.visible = false
@status_window.item = nil
@help_window.set_text("")
return
end
if Input.trigger?(Input::C)
@item = @buy_window.item
number = $game_party.item_number(@item)
if $game_switches[JFace::Discount::CostSwitch]
price = @item.price*$game_variables[JFace::Discount::CostAdjust]
price = price/100
if @item == nil or price > $game_party.gold or number == 99
Sound.play_buzzer
else
Sound.play_decision
max = @item.price == 0 ? 99 : $game_party.gold / @item.price
max = [max, 99 - number].min
@buy_window.active = false
@buy_window.visible = false
price = @item.price*$game_variables[JFace::Discount::CostAdjust]
price = price/100
@number_window.set(@item, max, price)
@number_window.active = true
@number_window.visible = true
end
else
if @item == nil or @item.price > $game_party.gold or number == 99
Sound.play_buzzer
else
Sound.play_decision
max = @item.price == 0 ? 99 : $game_party.gold / @item.price
max = [max, 99 - number].min
@buy_window.active = false
@buy_window.visible = false
@number_window.set(@item, max, @item.price)
@number_window.active = true
@number_window.visible = true
end
end
end
end
#--------------------------------------------------------------------------
# * Update Sell Item Selection
#--------------------------------------------------------------------------
def update_sell_selection
if Input.trigger?(Input::B)
Sound.play_cancel
@command_window.active = true
@dummy_window.visible = true
@sell_window.active = false
@sell_window.visible = false
@status_window.item = nil
@help_window.set_text("")
elsif Input.trigger?(Input::C)
@item = @sell_window.item
@status_window.item = @item
if @item == nil or @item.price == 0
Sound.play_buzzer
else
Sound.play_decision
max = $game_party.item_number(@item)
@sell_window.active = false
@sell_window.visible = false
depreciation = @item.price*$game_variables[JFace::Discount::SaleAdjust]
depreciation = depreciation/100
if JFace::Discount::DiscountSale == true
price = @item.price*$game_variables[JFace::Discount::CostAdjust]
price = price/100
@number_window.set(@item, max, depreciation)
else
@number_window.set(@item, max, depreciation)
end
@number_window.active = true
@number_window.visible = true
@status_window.visible = true
end
end
end
#--------------------------------------------------------------------------
# * Confirm Number Input
#--------------------------------------------------------------------------
def decide_number_input
Sound.play_shop
@number_window.active = false
@number_window.visible = false
case @command_window.index
when 0 # Buy
if $game_switches[JFace::Discount::CostSwitch]
price = @item.price*$game_variables[JFace::Discount::CostAdjust]
price = price/100
$game_party.lose_gold(@number_window.number * price)
else
$game_party.lose_gold(@number_window.number * @item.price)
end
$game_party.gain_item(@item, @number_window.number)
@gold_window.refresh
@buy_window.refresh
@status_window.refresh
@buy_window.active = true
@buy_window.visible = true
when 1 # sell
depreciation = @item.price*$game_variables[JFace::Discount::SaleAdjust]
depreciation = depreciation/100
if JFace::Discount::DiscountSale == true
price = @item.price*$game_variables[JFace::Discount::CostAdjust]
price = price/100
$game_party.gain_gold(@number_window.number * depreciation)
else
$game_party.gain_gold(@number_window.number * depreciation)
end
$game_party.lose_item(@item, @number_window.number)
@gold_window.refresh
@sell_window.refresh
@status_window.refresh
@sell_window.active = true
@sell_window.visible = true
@status_window.visible = false
end
end
end